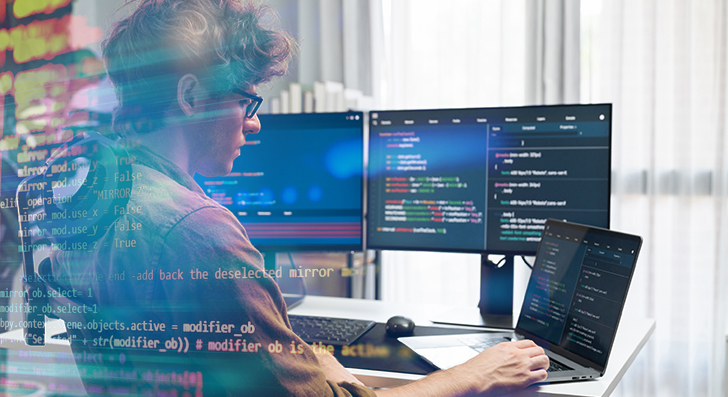
Scalability means your application can deal with growth—extra end users, a lot more data, and more targeted visitors—devoid of breaking. Like a developer, constructing with scalability in mind will save time and tension afterwards. Listed here’s a clear and realistic guidebook to help you get started by Gustavo Woltmann.
Design for Scalability from the beginning
Scalability isn't a thing you bolt on later—it ought to be component of your respective strategy from the start. A lot of applications fall short when they increase quick mainly because the original layout can’t handle the additional load. As being a developer, you'll want to Believe early regarding how your procedure will behave under pressure.
Start out by designing your architecture to get adaptable. Keep away from monolithic codebases where almost everything is tightly related. Rather, use modular layout or microservices. These styles crack your app into more compact, impartial pieces. Every module or provider can scale By itself with out impacting The full system.
Also, take into consideration your databases from day a single. Will it will need to take care of a million consumers or maybe 100? Choose the correct variety—relational or NoSQL—based on how your information will increase. System for sharding, indexing, and backups early, Even when you don’t need them however.
Yet another crucial position is to stop hardcoding assumptions. Don’t create code that only operates below existing problems. Contemplate what would transpire If the person foundation doubled tomorrow. Would your application crash? Would the databases decelerate?
Use style and design styles that guidance scaling, like concept queues or function-driven programs. These aid your app take care of far more requests with no receiving overloaded.
Once you Construct with scalability in mind, you are not just making ready for achievement—you are decreasing foreseeable future head aches. A nicely-prepared process is simpler to maintain, adapt, and grow. It’s improved to get ready early than to rebuild later on.
Use the best Database
Choosing the suitable database is really a key Element of making scalable apps. Not all databases are created the identical, and using the Incorrect you can sluggish you down or perhaps induce failures as your application grows.
Start by being familiar with your knowledge. Is it remarkably structured, like rows inside of a table? If Certainly, a relational database like PostgreSQL or MySQL is a good healthy. They're strong with associations, transactions, and regularity. Additionally they support scaling techniques like read through replicas, indexing, and partitioning to handle additional visitors and facts.
Should your details is more versatile—like user action logs, item catalogs, or files—think about a NoSQL selection like MongoDB, Cassandra, or DynamoDB. NoSQL databases are much better at dealing with significant volumes of unstructured or semi-structured info and might scale horizontally more quickly.
Also, consider your browse and compose designs. Are you carrying out numerous reads with much less writes? Use caching and read replicas. Do you think you're managing a heavy compose load? Look into databases that will cope with superior create throughput, as well as celebration-centered information storage techniques like Apache Kafka (for momentary details streams).
It’s also smart to Consider in advance. You might not have to have advanced scaling attributes now, but selecting a database that supports them signifies you gained’t will need to modify afterwards.
Use indexing to speed up queries. Avoid pointless joins. Normalize or denormalize your info according to your entry designs. And often keep an eye on databases effectiveness when you improve.
In brief, the correct database depends upon your app’s structure, velocity requires, And exactly how you be expecting it to improve. Just take time to choose sensibly—it’ll help save a great deal of difficulties later on.
Optimize Code and Queries
Fast code is essential to scalability. As your application grows, every single tiny delay provides up. Poorly penned code or unoptimized queries can decelerate functionality and overload your program. That’s why it’s vital that you Develop economical logic from the beginning.
Commence by creating clean up, uncomplicated code. Keep away from repeating logic and take away anything at all pointless. Don’t pick the most intricate Remedy if a straightforward just one operates. Keep your capabilities quick, focused, and simple to check. Use profiling resources to find bottlenecks—sites the place your code will take too extensive to run or uses an excessive amount memory.
Subsequent, evaluate your databases queries. These usually gradual items down much more than the code by itself. Be certain Each and every question only asks for the data you truly require. Prevent Choose *, which fetches anything, and as a substitute select distinct fields. Use indexes to hurry up lookups. And steer clear of executing too many joins, Specially throughout big tables.
In case you notice the identical facts currently being asked for again and again, use caching. Retailer the effects temporarily making use of instruments like Redis or Memcached so you don’t must repeat high priced operations.
Also, batch your database operations if you can. Rather than updating a row one by one, update them in groups. This cuts down on overhead and would make your application more effective.
Remember to examination with massive datasets. Code and queries that do the job fine with 100 records may well crash every time they have to take care of 1 million.
In short, scalable apps are quick apps. Keep your code tight, your queries lean, and use caching when necessary. These methods support your software keep clean and responsive, whilst the load improves.
Leverage Load Balancing and Caching
As your application grows, it's got to take care of extra users and more visitors. If every thing goes via 1 server, it'll rapidly become a bottleneck. That’s where load balancing and caching are available in. These two tools help keep the application rapidly, steady, and scalable.
Load balancing spreads incoming visitors across various servers. In lieu of one server accomplishing the many get the job done, the load balancer routes end users to unique servers determined by availability. This implies no single server receives overloaded. If one particular server goes down, the load balancer can deliver visitors to the Many others. Equipment like Nginx, HAProxy, or cloud-primarily based solutions from AWS and Google Cloud make this straightforward to put in place.
Caching is about storing information quickly so it can be reused immediately. When end users request the exact same data once more—like an item website page or perhaps a profile—you don’t really need to fetch it from your database whenever. You are able to provide it from your cache.
There are two popular forms of caching:
one. Server-aspect caching (like Redis or Memcached) suppliers knowledge in memory for fast entry.
2. Consumer-facet caching (like browser caching or CDN caching) retailers static data files close to the person.
Caching minimizes databases load, enhances velocity, and tends to make your application more productive.
Use caching for things which don’t modify normally. And often ensure that your cache is updated when facts does alter.
In a nutshell, load balancing and caching are simple but effective tools. Collectively, they assist your app manage additional customers, remain rapid, and recover from difficulties. If you intend to mature, you'll need equally.
Use Cloud and Container Applications
To build scalable programs, you would like tools that allow your app improve conveniently. That’s where cloud platforms and containers are available in. They provide you overall flexibility, lower set up time, and make scaling much smoother.
Cloud platforms like Amazon Internet Providers (AWS), Google Cloud Platform (GCP), and Microsoft Azure Enable you to lease servers and expert services as you'll need them. You don’t must get components or guess foreseeable future ability. When website traffic improves, you could increase more resources with just a few clicks or automatically using auto-scaling. When traffic drops, you can scale down to save money.
These platforms also give providers like managed databases, storage, load balancing, and safety resources. You are able to concentrate on building your application in lieu of taking care of infrastructure.
Containers are A different essential Device. A container packages your app and everything it really should operate—code, libraries, settings—into one device. This can make it uncomplicated to move your app concerning environments, from the laptop computer to the cloud, without the need of surprises. Docker is the preferred Instrument for this.
When your application makes use of numerous containers, applications like Kubernetes allow you to control them. Kubernetes handles deployment, scaling, and Restoration. If just one element of one's application crashes, it restarts it routinely.
Containers also allow it to be straightforward to individual parts of your application into solutions. You could update or scale areas independently, that is perfect for overall performance and trustworthiness.
In brief, applying cloud and container equipment means it is possible to scale fast, deploy quickly, and Recuperate immediately when troubles happen. If you need your application to expand without the need of limits, start out using these equipment early. They help you save time, minimize possibility, and assist you to remain centered on building, not repairing.
Watch Everything
Should you don’t monitor your application, you gained’t know when matters go Incorrect. Monitoring can help the thing is how your application is carrying out, place difficulties early, and make better choices as your app grows. It’s a critical part of developing scalable techniques.
Start out by monitoring basic metrics like CPU usage, memory, disk Area, and reaction time. These show you how your servers and products and services are performing. Resources like Prometheus, Grafana, Datadog, or New Relic can assist you accumulate and visualize this facts.
Don’t just observe your servers—monitor your app too. Keep an eye on how long it will take for consumers to load webpages, how often mistakes take place, and in which they arise. Logging more info equipment like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly can assist you see what’s taking place within your code.
Arrange alerts for vital complications. Such as, In the event your reaction time goes earlier mentioned a Restrict or even a support goes down, you ought to get notified instantly. This assists you fix issues speedy, generally in advance of end users even recognize.
Monitoring is usually handy any time you make alterations. Should you deploy a fresh characteristic and find out a spike in glitches or slowdowns, it is possible to roll it back right before it will cause actual harm.
As your application grows, targeted traffic and information maximize. Devoid of monitoring, you’ll pass up signs of trouble until eventually it’s also late. But with the right instruments in place, you continue to be in control.
To put it briefly, checking helps you maintain your app reliable and scalable. It’s not almost spotting failures—it’s about knowledge your method and ensuring that it works very well, even under pressure.
Closing Thoughts
Scalability isn’t only for big firms. Even little applications need a powerful Basis. By developing diligently, optimizing properly, and utilizing the right instruments, you can Create applications that develop efficiently without breaking stressed. Start tiny, Imagine large, and Create good.